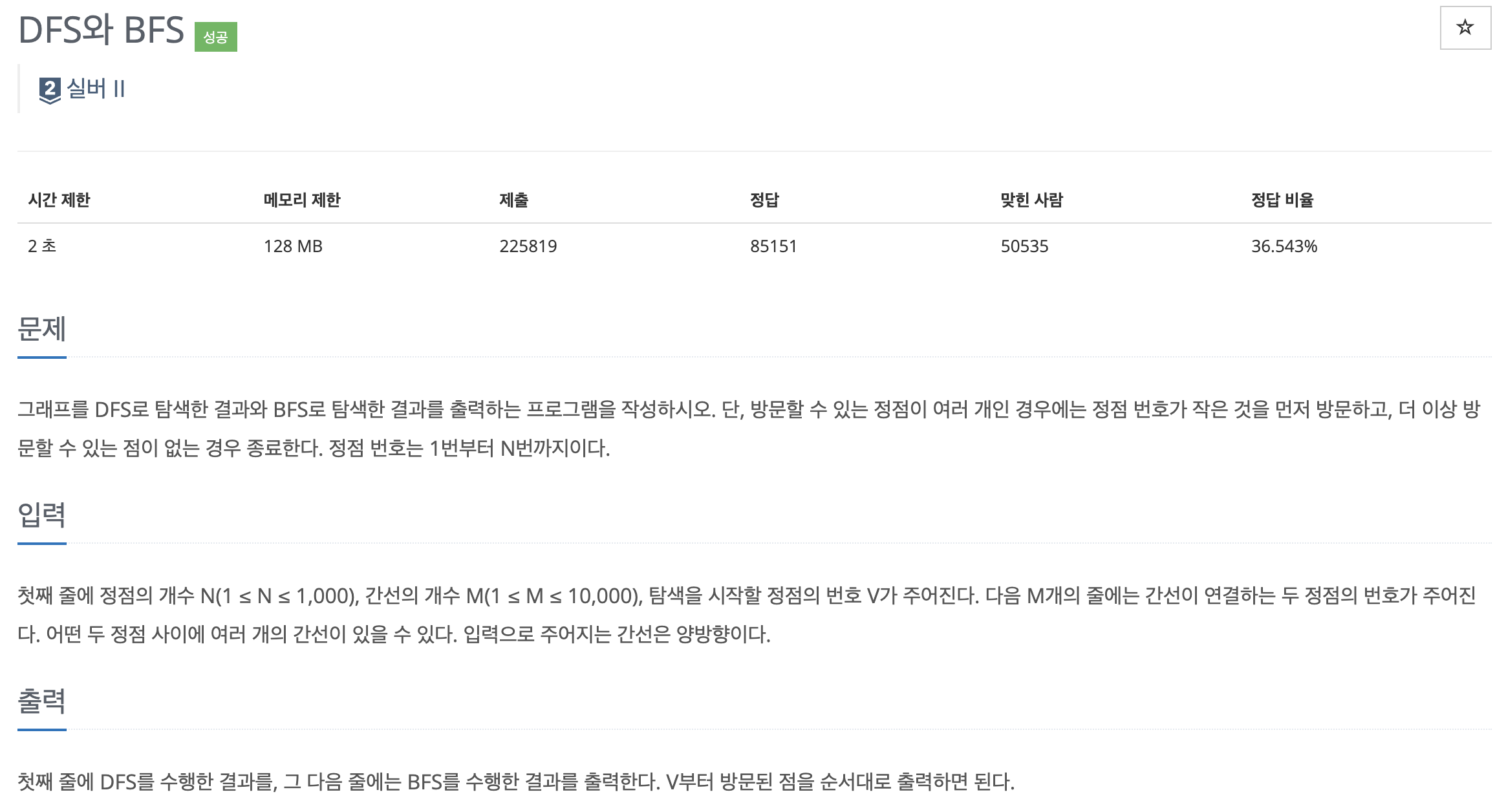
import Foundation
let NMV = readLine()!.components(separatedBy: " ").map { Int($0)! }
let N = NMV[0]
let M = NMV[1]
let V = NMV[2]
var graph = [String: [String]]()
initializeGraph()
for _ in 1...M {
let AB = readLine()!.components(separatedBy: " ")
let A = AB[0]
let B = AB[1]
graph[A]?.append(B)
graph[B]?.append(A)
}
printArray(DFS(start: String(V)))
printArray(BFS(start: String(V)))
func DFS(start: String) -> [String] {
var visitedQueue: [String] = []
var needVisitStack: [String] = [start]
while !needVisitStack.isEmpty {
let node: String = needVisitStack.removeLast()
if visitedQueue.contains(node) { continue }
visitedQueue.append(node)
// ์คํ์ removeLast()๋ก ๋
ธ๋๋ฅผ ๊ฐ์ ธ์ค๊ธฐ ๋๋ฌธ์ ์ ๋ ฌ์ ๋ด๋ฆผ์ฐจ์์ผ๋ก ํด์ค์ผ ์์ ์๋ถํฐ ๋ฝํ
needVisitStack += graph[node]?.sorted(by: { Int($0)! > Int($1)! }) ?? []
}
return visitedQueue
}
func BFS(start: String) -> [String] {
var visitedQueue: [String] = []
var needVisitQueue: [String] = [start]
while !needVisitQueue.isEmpty {
let node: String = needVisitQueue.removeFirst()
if visitedQueue.contains(node) { continue }
visitedQueue.append(node)
needVisitQueue += graph[node]?.sorted(by: { Int($0)! < Int($1)! }) ?? []
}
return visitedQueue
}
func initializeGraph() {
for i in 1...N {
graph[String(i)] = [] // ๊ทธ๋ํ ์ด๊ธฐํ
}
}
func printArray(_ arr: [String]) {
for str in arr {
if str.hashValue == arr.last?.hashValue {
print(str)
} else {
print(str, terminator: " ")
}
}
}
๋ฐ์ํ
'๐ ์ฝํ > BOJ' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
[Swift] ๋ฐฑ์ค 1676 ํฉํ ๋ฆฌ์ผ 0์ ๊ฐ์ (0) | 2023.03.30 |
---|---|
[Swift] ๋ฐฑ์ค1012 ์ ๊ธฐ๋๋ฐฐ์ถ (0) | 2023.03.30 |
[Swift] ๋ฐฑ์ค1389 ์ผ๋น ๋ฒ ์ด์ปจ์ 6๋จ๊ณ ๋ฒ์น (0) | 2023.03.30 |
[Swift] ๋ฐฑ์ค 11650 ์ขํ ์ ๋ ฌํ๊ธฐ (0) | 2023.03.29 |
[Swift] ๋ฐฑ์ค 10866 ๋ฑ (0) | 2023.03.29 |
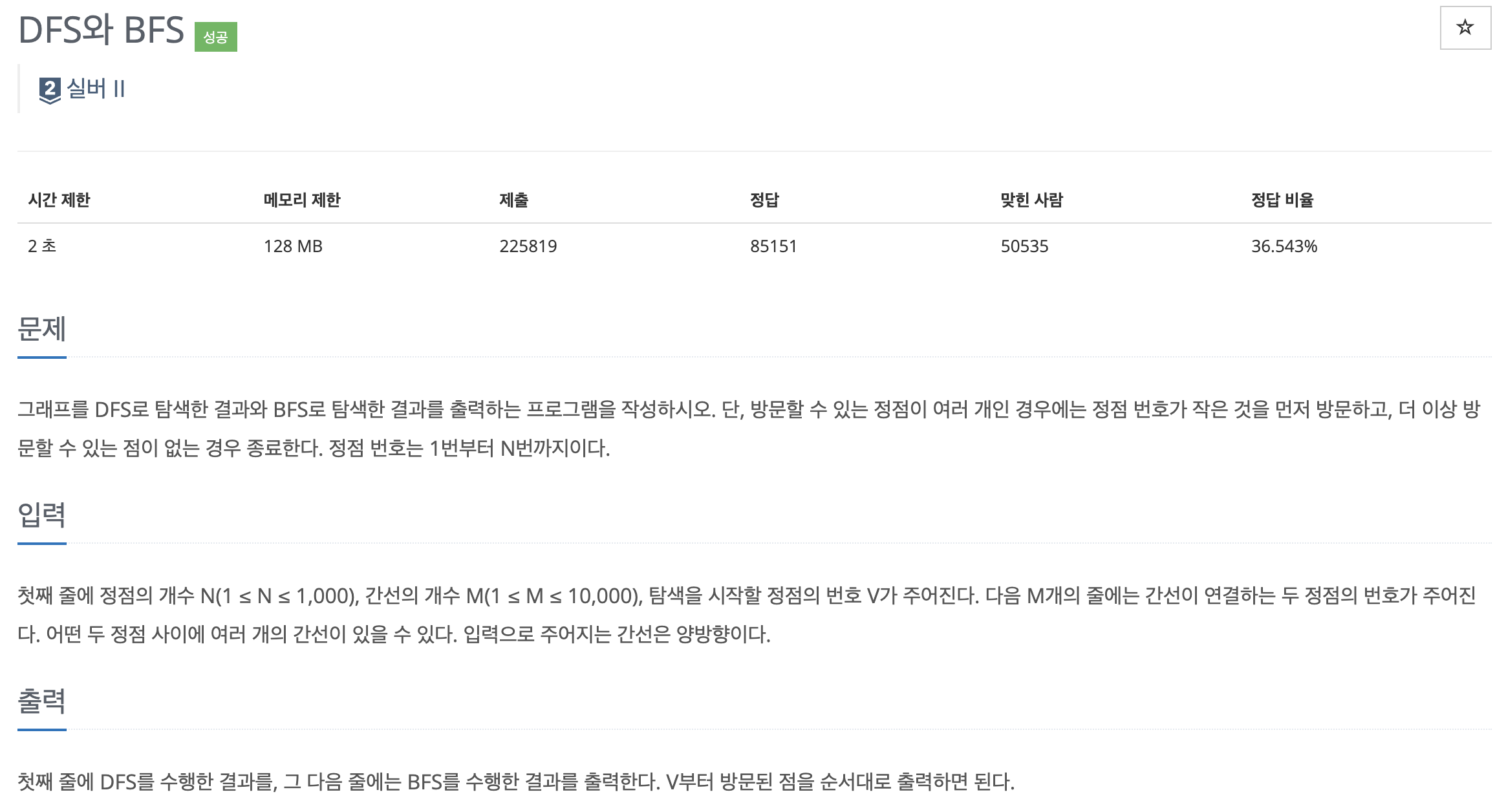
import Foundation
let NMV = readLine()!.components(separatedBy: " ").map { Int($0)! }
let N = NMV[0]
let M = NMV[1]
let V = NMV[2]
var graph = [String: [String]]()
initializeGraph()
for _ in 1...M {
let AB = readLine()!.components(separatedBy: " ")
let A = AB[0]
let B = AB[1]
graph[A]?.append(B)
graph[B]?.append(A)
}
printArray(DFS(start: String(V)))
printArray(BFS(start: String(V)))
func DFS(start: String) -> [String] {
var visitedQueue: [String] = []
var needVisitStack: [String] = [start]
while !needVisitStack.isEmpty {
let node: String = needVisitStack.removeLast()
if visitedQueue.contains(node) { continue }
visitedQueue.append(node)
// ์คํ์ removeLast()๋ก ๋
ธ๋๋ฅผ ๊ฐ์ ธ์ค๊ธฐ ๋๋ฌธ์ ์ ๋ ฌ์ ๋ด๋ฆผ์ฐจ์์ผ๋ก ํด์ค์ผ ์์ ์๋ถํฐ ๋ฝํ
needVisitStack += graph[node]?.sorted(by: { Int($0)! > Int($1)! }) ?? []
}
return visitedQueue
}
func BFS(start: String) -> [String] {
var visitedQueue: [String] = []
var needVisitQueue: [String] = [start]
while !needVisitQueue.isEmpty {
let node: String = needVisitQueue.removeFirst()
if visitedQueue.contains(node) { continue }
visitedQueue.append(node)
needVisitQueue += graph[node]?.sorted(by: { Int($0)! < Int($1)! }) ?? []
}
return visitedQueue
}
func initializeGraph() {
for i in 1...N {
graph[String(i)] = [] // ๊ทธ๋ํ ์ด๊ธฐํ
}
}
func printArray(_ arr: [String]) {
for str in arr {
if str.hashValue == arr.last?.hashValue {
print(str)
} else {
print(str, terminator: " ")
}
}
}
๋ฐ์ํ
'๐ ์ฝํ > BOJ' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
[Swift] ๋ฐฑ์ค 1676 ํฉํ ๋ฆฌ์ผ 0์ ๊ฐ์ (0) | 2023.03.30 |
---|---|
[Swift] ๋ฐฑ์ค1012 ์ ๊ธฐ๋๋ฐฐ์ถ (0) | 2023.03.30 |
[Swift] ๋ฐฑ์ค1389 ์ผ๋น ๋ฒ ์ด์ปจ์ 6๋จ๊ณ ๋ฒ์น (0) | 2023.03.30 |
[Swift] ๋ฐฑ์ค 11650 ์ขํ ์ ๋ ฌํ๊ธฐ (0) | 2023.03.29 |
[Swift] ๋ฐฑ์ค 10866 ๋ฑ (0) | 2023.03.29 |